DiffDRR
================
<!-- WARNING: THIS FILE WAS AUTOGENERATED! DO NOT EDIT! -->
> Auto-differentiable DRR synthesis and optimization in PyTorch
[](https://github.com/eigenvivek/DiffDRR/actions/workflows/test.yaml)
[](https://arxiv.org/abs/2208.12737)
[](LICENSE)
[](https://pepy.tech/project/diffdrr)
[](https://vivekg.dev/DiffDRR/)
[](https://github.com/psf/black)
`DiffDRR` is a PyTorch-based digitally reconstructed radiograph (DRR)
generator that provides
1. Auto-differentiable DRR syntheisis
2. GPU-accelerated rendering
3. A pure Python implementation
Most importantly, `DiffDRR` implements DRR synthesis as a PyTorch
module, making it interoperable in deep learning pipelines.
- [Installation Guide](#installation-guide)
- [Usage](#usage)
- [Example: Rigid 2D-to-3D
registration](#application-6-dof-slice-to-volume-registration)
- [How does `DiffDRR` work?](#how-does-diffdrr-work)
- [Citing `DiffDRR`](#citing-diffdrr)
## Installation Guide
To install `DiffDRR` with conda (or mamba):
``` zsh
conda install -c eigenvivek diffdrr
conda install -c nvidia pytorch-cuda=11.7 # Optional for GPU support
```
To install `DiffDRR` from PyPI:
``` zsh
pip install diffdrr
```
## Usage
The following minimal example specifies the geometry of the projectional
radiograph imaging system and traces rays through a CT volume:
``` python
import matplotlib.pyplot as plt
import torch
from diffdrr.drr import DRR
from diffdrr.data import load_example_ct
from diffdrr.visualization import plot_drr
# Read in the volume
volume, spacing = load_example_ct()
# Get parameters for the detector
bx, by, bz = torch.tensor(volume.shape) * torch.tensor(spacing) / 2
detector_kwargs = {
"sdr" : 300.0,
"theta" : torch.pi,
"phi" : 0,
"gamma" : torch.pi / 2,
"bx" : bx,
"by" : by,
"bz" : bz,
}
# Make the DRR
drr = DRR(volume, spacing, height=200, delx=4.0).to("cuda" if torch.cuda.is_available() else "cpu")
img = drr.project(**detector_kwargs)
ax = plot_drr(img)
plt.show()
```

On a single NVIDIA RTX 2080 Ti GPU, producing such an image takes
<details>
<summary>Code</summary>
``` python
```
</details>
34.9 ms ± 22.5 µs per loop (mean ± std. dev. of 7 runs, 10 loops each)
The full example is available at
[`tutorials/introduction.ipynb`](tutorials/introduction.ipynb).
## Application: 6-DoF Slice-to-Volume Registration
We demonstrate the utility of our auto-differentiable DRR generator by
solving a 6-DoF registration problem with gradient-based optimization.
Here, we generate two DRRs:
1. A fixed DRR from a set of ground truth parameters
2. A moving DRR from randomly initialized parameters
To solve the registration problem, we use gradient descent to maximize
an image loss similarity metric between the two DRRs. This produces
optimization runs like this:
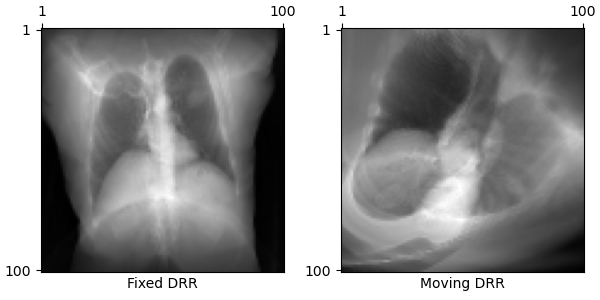
The full example is available at
[`experiments/registration`](experiments/registration).
## How does `DiffDRR` work?
`DiffDRR` reformulates Siddon’s method ([Siddon RL. Fast calculation of
the exact radiological path for a three-dimensional CT array. Medical
Physics, 2(12):252–5, 1985.](https://doi.org/10.1118/1.595715)), the
canonical algorithm for calculating the radiologic path of an X-ray
through a volume, as a series of vectorized tensor operations. This
version of the algorithm is easily implemented in tensor algebra
libraries like PyTorch to achieve a fast auto-differentiable DRR
generator.
## Citing `DiffDRR`
If you find `DiffDRR` useful in your work, please cite our
[paper](https://doi.org/10.1007/978-3-031-23179-7_1) (or the [freely
accessible arXiv version](https://arxiv.org/abs/2208.12737)):
@inproceedings{gopalakrishnanDiffDRR2022,
author = {Gopalakrishnan, Vivek and Golland, Polina},
title = {Fast Auto-Differentiable Digitally Reconstructed Radiographs for Solving Inverse Problems in Intraoperative Imaging},
year = {2022},
booktitle = {Clinical Image-based Procedures: 11th International Workshop, CLIP 2022, Held in Conjunction with MICCAI 2022, Singapore, Proceedings},
series = {Lecture Notes in Computer Science},
publisher = {Springer},
doi = {https://doi.org/10.1007/978-3-031-23179-7_1},
}