[](https://www.npmjs.org/package/command-line-commands)
[](https://www.npmjs.org/package/command-line-commands)
[](https://travis-ci.org/75lb/command-line-commands)
[](https://coveralls.io/github/75lb/command-line-commands?branch=master)
[](https://david-dm.org/75lb/command-line-commands)
[](https://github.com/feross/standard)
# command-line-commands
A lightweight module to help build a git-like command interface for your app.
Its job is to extract the command (the first argument, unless it's an option), check it's valid and either return it or throw. From there, you can parse the remaining args using your preferred option parser (e.g. [command-line-args](https://github.com/75lb/command-line-args), [minimist](https://github.com/substack/minimist) etc.).
## Synopsis
Create a list of valid commands (`null` represents "no command"). Supply it to `commandLineCommands()`, receiving back an object with two properties: `command` (the supplied command) and `argv` (the remainder of the command line args):
```js
const commandLineCommands = require('command-line-commands')
const validCommands = [ null, 'clean', 'update', 'install' ]
const { command, argv } = commandLineCommands(validCommands)
/* print the command and remaining command-line args */
console.log('command: %s', command)
console.log('argv: %s', JSON.stringify(argv))
```
We'll assume the above script is installed as `example`. Since the `validCommands` list includes `null`, running it without a command is valid:
```
$ example
command: null
argv: []
```
Running `example` with no command and one option:
```
$ example --verbose
command: null
argv: ["--verbose"]
```
Running `example` with both a command and an option:
```
$ example install --save something
command: install
argv: ["--save","something"]
```
Running `example` without a valid command will cause `commandLineCommands()` to throw.
From here, you can make a decision how to proceed based on the `command` and `argv` received. For example, if no command (`null`) was passed, you could parse the remaining `argv` for general options (in this case using [command-line-args](https://github.com/75lb/command-line-args)):
```js
if (command === null) {
const commandLineArgs = require('command-line-args')
const optionDefinitions = [
{ name: 'version', type: Boolean }
]
// pass in the `argv` returned by `commandLineCommands()`
const options = commandLineArgs(optionDefinitions, { argv })
if (options.version) {
console.log('version 1.0.1')
}
}
```
The same example, using [minimist](https://github.com/substack/minimist):
```js
if (command === null) {
const minimist = require('minimist')
// pass in the `argv` returned by `commandLineCommands()``
const options = minimist(argv)
if (options.version) {
console.log('version 1.0.1')
}
}
```
## More examples
Both examples use [command-line-args](https://github.com/75lb/command-line-args) for option-parsing.
- [Simple](https://github.com/75lb/command-line-commands/blob/master/example/simple.js): A basic app with a couple of commands.
- [Advanced](https://github.com/75lb/command-line-commands/blob/master/example/advanced/git.js): A more complete example, implementing part of the git command interface.
## Usage guides
Usage guides can be generated by [command-line-usage](https://github.com/75lb/command-line-usage). Here is a simple example ([code](https://github.com/75lb/command-line-commands/blob/master/example/usage.js)):
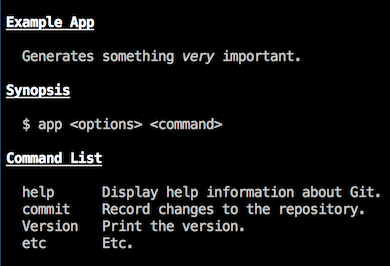
# API Reference
**Example**
```js
const commandLineCommands = require('command-line-commands')
```
<a name="exp_module_command-line-commands--commandLineCommands"></a>
### commandLineCommands(commands, [argv]) ⇒ <code>Object</code> ⏏
Parses the `argv` value supplied (or `process.argv` by default), extracting and returning the `command` and remainder of `argv`. The command will be the first value in the `argv` array unless it is an option (e.g. `--help`).
**Kind**: Exported function
**Throws**:
- `INVALID_COMMAND` - user supplied a command not specified in `commands`.
| Param | Type | Description |
| --- | --- | --- |
| commands | <code>string</code> \| <code>Array.<string></code> | One or more command strings, one of which the user must supply. Include `null` to represent "no command" (effectively making a command optional). |
| [argv] | <code>Array.<string></code> | An argv array, defaults to the global `process.argv` if not supplied. |
* * *
© 2015-21 Lloyd Brookes \<75pound@gmail.com\>. Documented by [jsdoc-to-markdown](https://github.com/jsdoc2md/jsdoc-to-markdown).